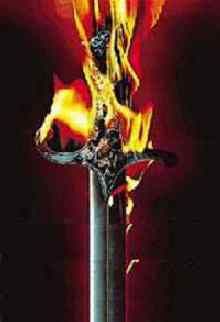 |
Śmieci, śmieci i śmieci Nasze-śmieci.pl
|
Zobacz poprzedni temat :: Zobacz następny temat |
Autor |
Wiadomość |
dfkld
Gość
|
Wysłany: Pią 23:49, 06 Lis 2009 Temat postu: lpd |
|
|
Kod: |
#======================================================================
# ** Keyboard Input Module (Revised)
#------------------------------------------------------------------------------
# by DerVVulfman
# version 1.2
# 08-15-2007
#------------------------------------------------------------------------------
# Based on...
# Keyboard Input Module version 3
# by Near Fantastica (06.07.05)
#==============================================================================
module Input
#--------------------------------------------------------------------------
# * Get Current Keypress State (Regular presses)
#--------------------------------------------------------------------------
def Input.getstate(key)
return true unless Win32API.new("user32","GetKeyState",["i"],"i").call(key).between?(0, 1)
return false
end
#--------------------------------------------------------------------------
# * Get Continuous Keyboard State (CapsLock, NumLock, ScrollLock)
#--------------------------------------------------------------------------
def Input.keyboardstate(rkey, key = 0)
return false unless Win32API.new("user32","GetKeyState",['i'],'i').call(rkey) & 0x01 == key
return true
end
#--------------------------------------------------------------------------
# * Numberpad Input System
#--------------------------------------------------------------------------
def Input.get_direction
return "Center" if Input.getstate(12)
return "PgUp" if Input.getstate(33)
return "PgDn" if Input.getstate(34)
return "End" if Input.getstate(35)
return "Home" if Input.getstate(36)
return "Left" if Input.getstate(37)
return "Up" if Input.getstate(38)
return "Right" if Input.getstate(39)
return "Down" if Input.getstate(40)
return "Ins" if Input.getstate(45)
return "Del" if Input.getstate(46)
return "*" if Input.getstate(106)
return "+" if Input.getstate(107)
return "-" if Input.getstate(109)
return "/" if Input.getstate(111)
if Input.keyboardstate(144,1)
for key in 96..105 #ASCII KEYS 48(0) to 57(9)
if Input.getstate(key)
return (key-48).chr
end
end
end
return nil
end
#--------------------------------------------------------------------------
# * Function Keys (Tab / Enter / Ctrl / Etc)
#--------------------------------------------------------------------------
def Input.get_function
return "Back" if Input.getstate(8)
if Input.getstate(16)
return "BackTab" if Input.getstate(9)
else
return "Tab" if Input.getstate(9)
end
return "Enter" if Input.getstate(13)
return "Ctrl" if Input.getstate(17)
return "Alt" if Input.getstate(18)
return "Pause" if Input.getstate(19)
return "Esc" if Input.getstate(27)
return "PrntScr" if Input.getstate(44)
# Function Keys (F1 - F12)
for key in 112..123
if Input.getstate(key)
num = key - 111
return "F" + num.to_s
end
end
return nil
end
#--------------------------------------------------------------------------
# * Individual Keys (Left OR Right keys, and Windows & Application keys)
#--------------------------------------------------------------------------
def Input.get_individual
return "CapsLock" if Input.getstate(20)
return "NumLock" if Input.getstate(144)
return "ScrlLock" if Input.getstate(145)
return "Lf Win" if Input.getstate(91)
return "Rt Win" if Input.getstate(92)
return "App Key" if Input.getstate(93)
return "Lf Shift" if Input.getstate(160)
return "Rt Shift" if Input.getstate(161)
return "Lf Ctrl" if Input.getstate(162)
return "Rt Ctrl" if Input.getstate(163)
return "Lf Alt" if Input.getstate(164)
return "Rt Alt" if Input.getstate(165)
return nil
end
#--------------------------------------------------------------------------
# * Letter Keys (Just your regular alphabet, caps or otherwise)
#--------------------------------------------------------------------------
def Input.get_letters
for key in 65..90 #ASCII KEYS 65(a) to 90(z)
if Input.getstate(key)
# Ensure lower-case if NEITHER or BOTH the shift and caps are pressed
if (Input.getstate(16) and Input.keyboardstate(20,1) ) or
(Input.getstate(16) != true and Input.keyboardstate(20,1) != true)
return key.chr.downcase
else
# Otherwise, uppercase
return key.chr.upcase
end
end
end
return nil
end
#--------------------------------------------------------------------------
# * Number Keys (only the top keys)
#--------------------------------------------------------------------------
def Input.get_numbers
return nil if Input.getstate(16)
for key in 48..57 #ASCII KEYS 48(0) to 57(9)
if Input.getstate(key)
return key.chr
end
end
return nil
end
#--------------------------------------------------------------------------
# * Keys (All others)
#--------------------------------------------------------------------------
def Input.get_key
if Input.getstate(32)
return " "
end
# Ensure lower-case if NEITHER or BOTH the shift and caps are pressed
if (Input.getstate(16) and Input.keyboardstate(20,1) ) or
(Input.getstate(16) != true and Input.keyboardstate(20,1) != true)
return ";" if Input.getstate(186)
return "=" if Input.getstate(187)
return "," if Input.getstate(188)
return "-" if Input.getstate(189)
return "." if Input.getstate(190)
return "/" if Input.getstate(191)
return "`" if Input.getstate(192)
return "[" if Input.getstate(219)
return "\\" if Input.getstate(220)
return "]" if Input.getstate(221)
return "'" if Input.getstate(222)
# Otherwise, uppercase
else
return ":" if Input.getstate(186)
return "+" if Input.getstate(187)
return "<" if Input.getstate(188)
return "_" if Input.getstate(189)
return ">" if Input.getstate(190)
return "?" if Input.getstate(191)
return "~" if Input.getstate(192)
return "{" if Input.getstate(219)
return "|" if Input.getstate(220)
return "}" if Input.getstate(221)
return "\x22" if Input.getstate(222)
end
# Shifted Top-row Numbers (not in ASCII order)
for key in 48..57
if Input.getstate(key) and Input.getstate(16)
case key
when 48; return ")"
when 49; return "!"
when 50; return "@"
when 51; return "#"
when 52; return "$"
when 53; return "%"
when 54; return "^"
when 55; return "&"
when 56; return "*"
when 57; return "("
end
end
end
return nil
end
end |
|
|
Powrót do góry |
|
 |
|
 |
|
|
Możesz pisać nowe tematy Możesz odpowiadać w tematach Nie możesz zmieniać swoich postów Nie możesz usuwać swoich postów Nie możesz głosować w ankietach
|
fora.pl - załóż własne forum dyskusyjne za darmo
Powered by phpBB © 2001, 2002 phpBB Group
|